The Single Responsibility Principle (SRP), the first of the SOLID design principles, states that each class should have one reason to change—in other words, one job. Below is a more detailed class diagram that illustrates how you might separate different responsibilities into their own classes.
Detailed Class Diagram
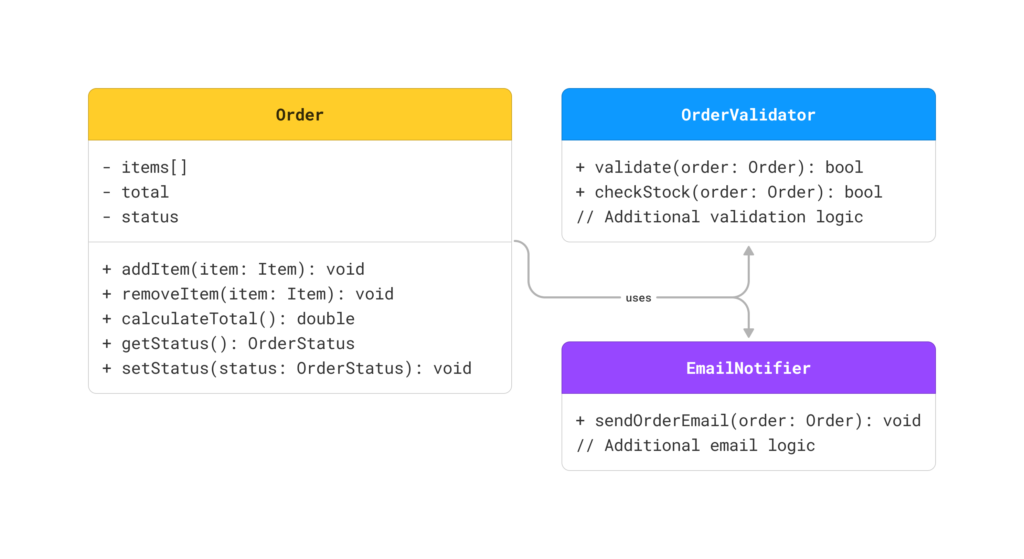
Order Class
- Purpose: Represents the data and basic operations for an order.
- Attributes:
- items: List<Item> – stores items in the order
- total: double – holds the total price
- status: OrderStatus – current status of the order (e.g., PENDING, SHIPPED)
- Methods:
- addItem(item: Item) – adds an item to the order
- removeItem(item: Item) – removes an item from the order
- calculateTotal() – updates and returns the total cost of the order
- getStatus(), setStatus(status: OrderStatus) – getters/setters for order status
Single Responsibility: The Order class only deals with managing order data (items, total, status). It does not handle validation or sending emails.
OrderValidator Class
- Purpose: Manages all validation logic related to an order.
- Methods:
- validate(order: Order): bool – checks if an order meets certain criteria (e.g., items are not empty, total is correct)
- checkStock(order: Order): bool – verifies item availability
- (Additional validation methods as needed)
Single Responsibility: The OrderValidator class is only responsible for validating the Order object. Changing validation rules means modifying only this class, leaving other classes untouched.
EmailNotifier Class
- Purpose: Handles email communication related to the order.
- Methods:
- sendOrderEmail(order: Order): void – sends a confirmation or status update email based on the order status
- Additional notification methods as needed)
Single Responsibility: The EmailNotifier class only deals with sending emails. If you change the email template or add new notification channels, you don’t have to modify Order or OrderValidator.
Why This Matters
- Maintainability: Smaller classes with single responsibilities are easier to debug, update, or enhance.
- Scalability: You can add or remove functionalities (e.g., new notification channels) without changing existing classes, preventing cascading breaks.
- Testability: Each class can be tested in isolation. Mock dependencies in unit tests to confirm validation logic or email-sending works as expected.
- Readability: Future contributors can quickly understand each class’s role, making collaboration and onboarding smoother.
Conclusion
Adopting the Single Responsibility Principle gives every class a clear, singular focus. In larger applications, this translates into organized, modular code that’s simpler to refactor and maintain over time. Remember, SRP is just the beginning of SOLID—but it’s often the most impactful in cleaning up unwieldy “god classes.”
Stay tuned for more insights on the rest of the SOLID principles, and feel free to reach out on LinkedIn to discuss how these principles can elevate your next project!