In the ever-evolving world of software development, writing clean, maintainable, and scalable code is essential. That’s where SOLID design principles come into play. Coined by Robert C. Martin (“Uncle Bob”), these five guidelines ensure your object-oriented code remains easy to extend, test, and refactor as requirements change.
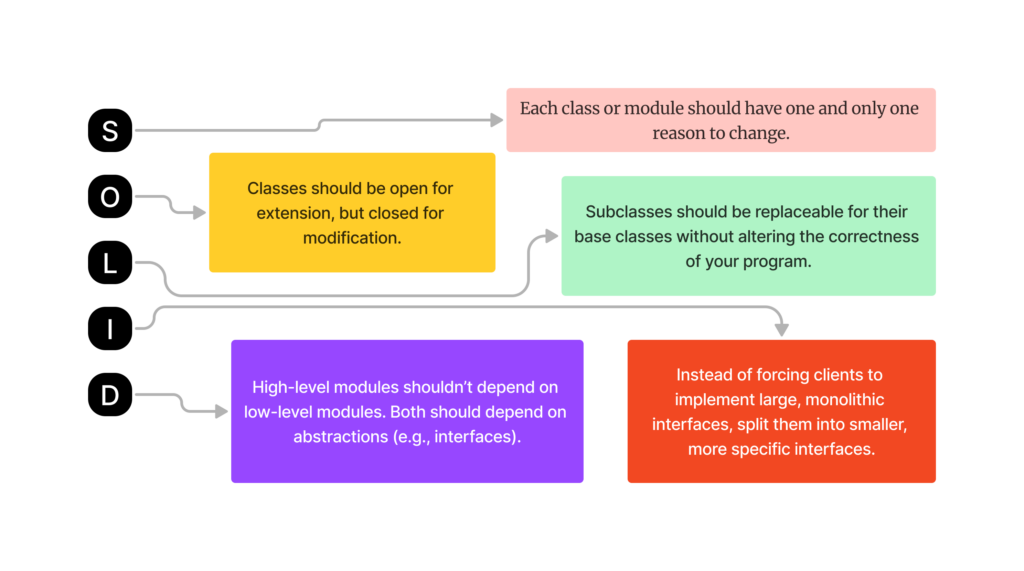
In this article, we’ll explore what SOLID means, why these principles matter, and how they enhance modern software engineering practices.
What Are the SOLID Design Principles?
1. Single Responsibility Principle (SRP)
Each class or module should have one and only one reason to change. This ensures your code remains focused, reducing complexity and avoiding unwanted side effects.
2. Open/Closed Principle (OCP)
Classes should be open for extension, but closed for modification. Instead of editing existing logic when adding new features, you extend the codebase in a way that doesn’t break existing functionality.
3. Liskov Substitution Principle (LSP)
Subclasses should be replaceable for their base classes without altering the correctness of your program. Essentially, an instance of a subclass should behave the same way as an instance of its parent class.
4. Interface Segregation Principle (ISP)
Instead of forcing clients to implement large, monolithic interfaces, split them into smaller, more specific interfaces. This way, classes only implement methods they actually need, reducing bloat and confusion.
5. Dependency Inversion Principle (DIP)
High-level modules shouldn’t depend on low-level modules. Both should depend on abstractions (e.g., interfaces). This makes your system more flexible, testable, and easier to maintain as you can swap out implementations with minimal changes to the high-level code.
Why Use SOLID Design Principles?
1. Maintainability
SOLID principles significantly reduce the complexity of your codebase. When each class or module has a single job (SRP) and relies on clear interfaces (ISP, DIP), it becomes much simpler to troubleshoot, refactor, or update features.
2. Scalability
As your application grows, you want to add new functionalities without breaking existing code. By following OCP, you can extend behaviors seamlessly. Meanwhile, LSP ensures that your hierarchy of classes integrates smoothly when new subclasses are introduced.
3. Testability
Writing unit tests becomes more straightforward when responsibilities are well-defined, and code depends on abstractions instead of concrete implementations. You can mock dependencies in tests with ease, ensuring that changes in one area won’t wreak havoc elsewhere.
4. Better Collaboration
In teams, code that follows SOLID principles is easier to understand and modify. New team members can quickly comprehend class responsibilities and interfaces, which speeds up onboarding and development.
5. Professional Credibility
Understanding and applying SOLID principles demonstrates a strong grasp of software engineering best practices. It shows that you can architect robust applications—a skill highly valued in tech interviews and on-the-job performance.
Practical Tips for Getting Started
• Refactor Step-by-Step: If you have an existing codebase, introduce SOLID principles incrementally. Focus on one principle at a time, such as extracting large interfaces into smaller ones or separating out a class’s responsibilities.
• Use Design Patterns: Many established design patterns (Factory, Observer, Strategy, etc.) are built with SOLID in mind. Recognize where these patterns can help implement or reinforce these principles.
• Review and Collaborate: Regular code reviews are an excellent opportunity to discuss SOLID concepts. Encourage your team to point out potential SRP or OCP violations, helping everyone learn.
Conclusion
By applying SOLID design principles, you’ll write software that’s more robust, flexible, and enjoyable to maintain—even as your applications grow in complexity. Whether you’re developing a small hobby project or leading a large-scale enterprise system, these guidelines serve as a foundation for clean code and professional engineering practices.
Which SOLID principle resonates most with your development style? Let me know on LinkedIn or drop me a direct message—I’d love to hear your perspective. Happy coding!